Memory segments
Introduced new memory segments feature:
- You can have up to 10 MB of additional memory by activating asynchronous memory segments.
- Each memory segment is a string with an ID from 0 to 99.
- Maximum segment data length is 100 KB.
- Segments are asynchronous, you should request them using
RawMemory.setActiveSegments
. The data will be available on the next tick.
- You cannot have more than 10 segments active at the same time.
- Active segments are available in
RawMemory.segments
object and are saved automatically.
- Activating and saving segments have no added CPU cost.
Example:
RawMemory.setActiveSegments([0,3]);
console.log(RawMemory.segments[0]);
console.log(RawMemory.segments[3]);
RawMemory.segments[3] = '{"foo": "bar", "counter": 15}';
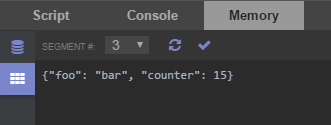
You can use this feature to store data which is not needed every tick, for example pathfinding caches, or collected statistics. Offloading data structures from Memory
to asynchronous segments will allow to save some CPU spent on Memory
parsing.
Other changes
-
You can now create a RoomVisual
object using the constructor without a roomName
parameter and post visuals to all rooms simultaneously:
new RoomVisual().circle(30,30, {fill: 'red'});
new RoomVisual('W1N1').rect(10,10,40,40);
This code will display both the circle and the rectangle in the room W1N1, and only the circle in any another room.
- Changed
RoomVisual.text
method style options:
- Renamed
size
option to font
. It can be either a number or a string in one of the following forms:
0.7
0.7 serif
bold italic 1.5 Times New Roman
20px sans-serif
- absolute size in pixels
- Added new
stroke
and strokeWidth
options.
- Added new
backgroundColor
and backgroundPadding
options.
Example:
new RoomVisual().text('Text 123', 10, 10, {
font: 'bold italic .7 serif',
color: 'white',
backgroundColor: '#88ff88',
backgroundPadding: .3,
stroke: '#005500',
strokeWidth: .15
});
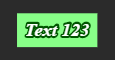
These features are supported in private server v2.4.0.